Posted on: 24 October 2020October 2020 Site Upgrades
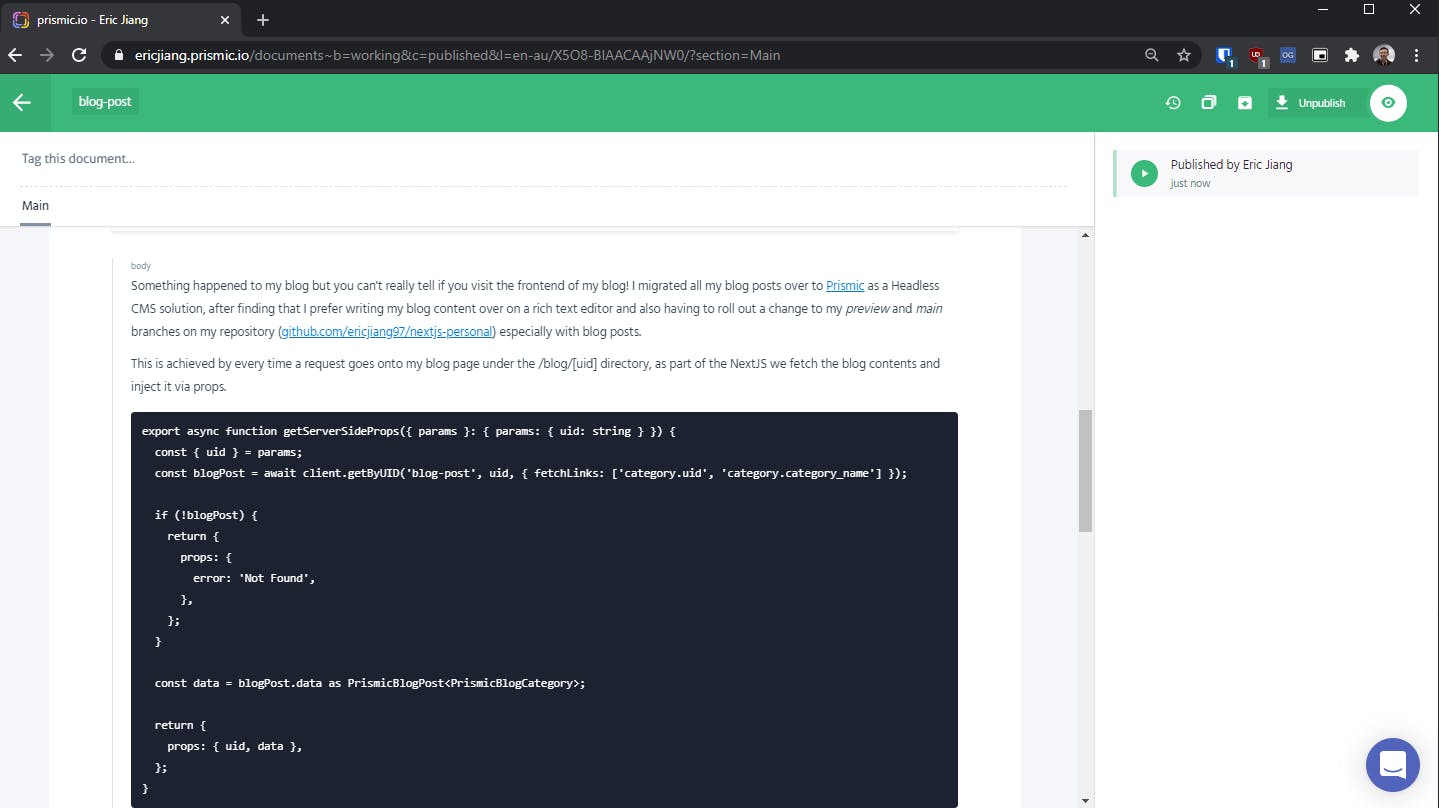
Something happened to my blog but you can't really tell if you visit the frontend of my blog! I migrated all my blog posts over to Prismic as a Headless CMS solution, after finding that I prefer writing my blog content over on a rich text editor and also having to roll out a change to my preview and main branches on my repository (github.com/ericjiang97/nextjs-personal) especially with blog posts.
This is achieved by every time a request goes onto my blog page under the /blog/[uid] directory, as part of the NextJS we fetch the blog contents and inject it via props.
export async function getServerSideProps({ params }: { params: { uid: string } }) { const { uid } = params; const blogPost = await client.getByUID('blog-post', uid, { fetchLinks: ['category.uid', 'category.category_name'] }); if (!blogPost) { return { props: { error: 'Not Found', }, }; } const data = blogPost.data as PrismicBlogPost<PrismicBlogCategory>; return { props: { uid, data }, }; }
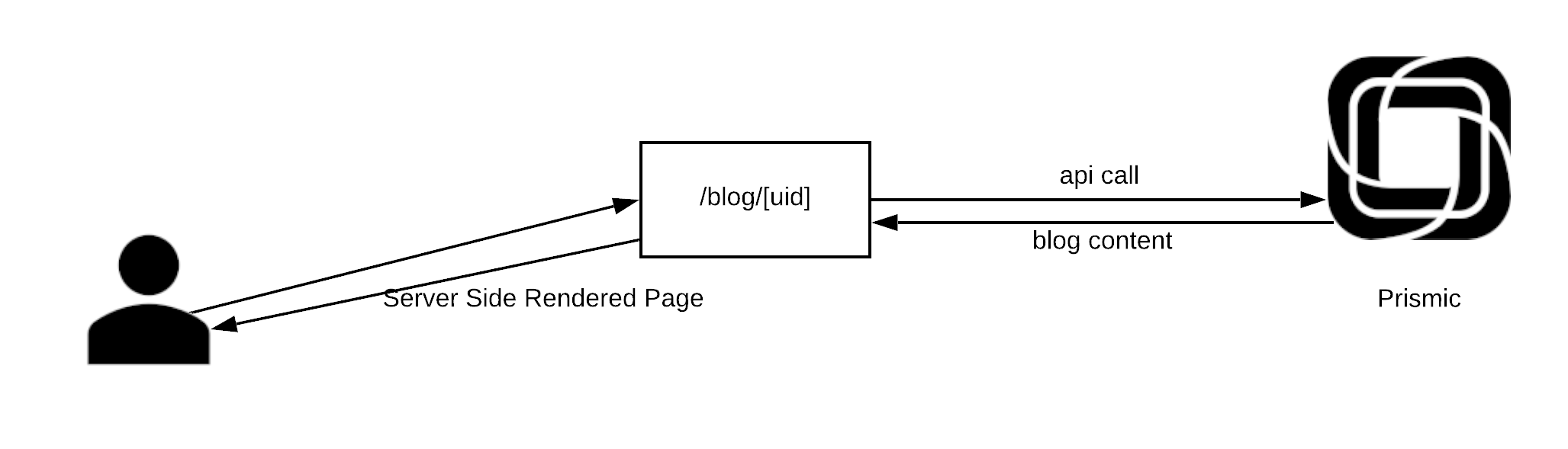
You also may have noticed that I also added some customised types in order to support TypeScript a bit better, especially with some typed interfaces
For example, this is my types files for my Prismic Blog Content
import { RichTextBlock } from 'prismic-reactjs'; export interface PrismicBlogPost<T> { title: RichTextBlock[]; author: RichTextBlock[]; preview: boolean; published_time: string; summary: string; body: any; banner?: { dimensions: { width: number; height: number }; alt: null; copyright: null; url: string; }; category: PrismicBlogPostCategory<T>; } export interface PrismicBlogPostCategory<T> { id: string; type: 'category'; tags: string[]; slug: string; lang: string; uid: string; link_type: 'Document'; isBroken: false; data: T; } export interface PrismicBlogCategory { id: string; category_name: string; }
You can check out the Prismic Blog Code here, please note that the Prismic API keys/secrets are provided as environment variables.
Amendment
In a following update to my site, I've changed the way blog posts are fetched from getServerSideProps to getStaticProps on my landing and blog index pages, this will allow faster rendering on these pages. A big thanks to Jane Machun Wong for the tip!
Since Next.js 9.4, you can make getStaticProps rerun per a period of time at max at runtime so it can generate new/modified pages after the build timehttps://t.co/3NJUoH595l pic.twitter.com/xOD4Ts247p
— Jane Manchun Wong (@wongmjane) October 26, 2020